I’ve spent the last year in the ‘uncanny valley’ of the Arduino. That’s the point where you understand the tutorials at Arduino.cc, but still don’t get much from the material on gitHub because trained programmers would never stoop to using the wire.h library when they could just roll their own in native C++ using the avr-g compiler. The problem with establishing sensor communication at the level of the TWI peripheral inside the AVR is that there are so many fiddling details to keep track of that it quickly overruns the 7±2 things this average human can hold in his head at one time: Computers aren’t the only things that crash after a buffer overflow! So this post is meant to be a chunking exercise for beginner-intermediate level people who want to get a new sensor working using the standard IDE. I’ve tried to distill it all down to things that I run into frequently, but there’s still a lot of material here: So pour yourself a cuppa before diving in...
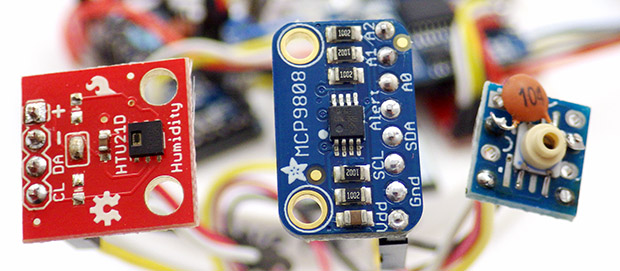
The great strength of I2C is that you can put so many sensors on the same four wires. But for units with several pre-made modules connected you might have to remove a few smd resistors from the breakouts, or the pull-up on the bus might become too aggressive. Most of the time I just leave them on, so I can extend the wire length, or crank up the bus clock…
REGISTERS are simply memory locations inside an I²C device. The summary of how many registers there are in a given sensor, and what they control or contain is called a register map. Most of the information on the sensor’s datasheet is about explaining how each register functions, and they can be quite a slog to read through because the information is rarely presented in an intuitive way.
To give you a sense of what I mean by that: take a look at page 14 of the manufacturers datasheet for the ADXL345 accelerometer:
Then take a look at the interactive register map for that sensor over at the i2cdevlib site:
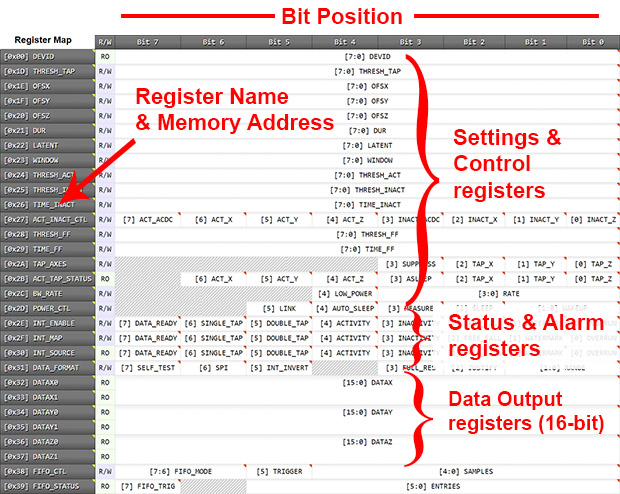
Even if you’ve never worked with registers before, jrowberg’s visual grid layout makes it easy to see how the sensor’s memory is divided into sections, which are doing different things.
There are many kinds of registers but for this introduction I am going to group them into three general types: Control, Data and Status registers, and provide brief examples of code that you can use to work with each of them. The functions named with the i2c_ prefix should be generic enough to work with most I²C sensors, but I’ll also be referring to a few specific cases to show how you might need to modify those basic functions.
1) Control Registers
Most sensors change how they operate based on the values stored in control registers. Think of control registers as banks of On/Off switches, which you turn on by setting a bit to 1 and turn off by setting that bit to 0. I²C chip-based sensors often have a dozen or more operational settings for things like bit-depth, sampling speed, noise reduction, etc., so you usually need to set bits in several different control registers before you can actually take a reading. And sometimes there are “special chip functions” that perform some kind of post processing on those sensor readings that would be hard to replicate on the Arduino. These can add an extra layer of control settings to take care of when you initialize the sensor.
Arduino’s wire library can only transfer 8-bit bytes over the I²C bus, so that’s the smallest amount of information you can write into a register memory location at one time. This can potentially change eight of those control switches simultaneously and, for parameters that are controlled by more than one bit, sometimes it’s actually required that you set them in one register-writing operation. Most people use byte variables for the sensor’s bus and register memory addresses, but once you’ve figured out the pattern you need to set up in control register switch-bits, it helps to write that information as a long form binary number (eg. 0b00001111) so you can see the on/off states when you read through your code.
Writing a byte to a sensor’s control register can be done with four basic steps:
Wire.beginTransmission(deviceAddress); // Attention sensor @ deviceAddress!
Wire.write(registerAddress); // command byte to target the register location
Wire.write(dataByte); // new data to put into that memory register
Wire.endTransmission();
The I²C deviceAddress is set by the manufacturer but some can be modified from their defaults by connecting solder pads on the breakout board. Since the bus address of a given sensor IC can vary from one module to the next I keep Rob Tillaart’s bus scanner handy to find them, and more importantly to discover when two sensors are fighting with each other by trying to use the same address on the bus. The registerAddress moves a pointer inside the chip to the memory location you specified. You can think of this pointer as a read/write head and once that pointer is aiming at a specific register, the next byte you send along the wires will over-write the data that was previously stored there.
The startup default values for a given control register are often a string of zeros because all the chip functions being controlled by that register are turned off. Unfortunately this means you’ll find lots of poorly commented code examples out there where people simply write zero into a control register without explaining which of the eight different functions they were aiming for because seven of those were still at their default zero-values anyway.
Reading data from a sensors memory register(s) requires two phases:
Wire.beginTransmission(deviceAddress); // get the sensors attention
Wire.write(registerAddress); // move your memory pointer to registerAddress
Wire.endTransmission(); // completes the ‘move memory pointer’ transaction
Wire.requestFrom(deviceAddress, 2); // send me the data from 2 registers
firstRegisterByte = Wire.read(); // byte from registerAddress
secondRegisterByte = Wire.read(); // byte from registerAddress +1
The first phase tells the I²C slave device which memory register that we want to read but we have complete the read operation in two separate steps because the wire library buffers everything behind the scenes and does not actually send anything until it gets the Wire.endTransmission(); command. The second phase is the data reading process and you can request as many bytes as you want with the second parameter in Wire.requestFrom . The memory location pointer inside the sensor increments forward automatically from the initial memory register address for each new byte that it sends. (The ‘dummy-write 1st’ method is similar to the procedure you’d use when doing a random data read from larger eeprom memory chips as well as sensors)
These simple patterns are at the heart of every I²C transaction, and since they are used so frequently, they often get bundled into their own functions:
byte i2c_readRegisterByte (uint8_t deviceAddress, uint8_t registerAddress) {
byte registerData;
byte i2c_writeRegisterByte (uint8_t deviceAddress, uint8_t registerAddress, uint8_t newRegisterByte) {
Wire.beginTransmission(deviceAddress);
Wire.write(registerAddress);
result = Wire.endTransmission(); // Wire.endTransmission(); returns 0 if write operation was successful
// delete this comment – it was only needed for blog layout.
//faulty, delete all the spaces and/or retype it slowly and carefully ensuring you enter only ASCII characters.
Those two functions will let you control the majority of the I²C sensors on the market, provided you can figure out the correct pattern of bits to send from the datasheet. A common strategy for keeping track of the multi-bit combinations that you want to load into your sensor control registers is to declare them with #define statements at the beginning of your program, which replace the human readable labels with the actual binary numbers at compile time.
For example the ADXL345 can range from 3 samples per second to 1600 samples per second, depending on four bits in the ADXL345_BW_RATE register. A set of define statements to represent those bit combinations might look like:
byte ADXL345_Address=0x53; // the sensors i2c bus address (as a hex number)
byte ADXL345_BW_RATE=0x2c; // the memory register address
#define ADXL345_BW_1600 0b00001111
#define ADXL345_BW_800 0b00001110
#define ADXL345_BW_400 0b00001101
#define ADXL345_BW_200 0b00001100
#define ADXL345_BW_100 0b00001011
#define ADXL345_BW_50 0b00001010
#define ADXL345_BW_25 0b00001001
#define ADXL345_BW_12 0b00001000
#define ADXL345_BW_6 0b00000111
#define ADXL345_BW_3 0b00000110
etc…. Note that all of these combinations assume normal power mode (bit4=0)
So a command to set the sampling rate to 50 Hz could be written as:
i2c_writeRegisterByte(ADXL345_Address, ADXL345_BW_RATE, ADXL345_BW_50);
The cool thing about using defines is that they do not use any ram memory like byte variables would. And you can usually find code examples on gitHub where someone has transcribed the entire register address list into a set of defines, which you can simply copy and paste into your own code. This saves you a great deal of time, though there’s always the chance they made a transcription error somewhere. Also note that typical datasheets & ‘c’ language examples express those numbers as hex “0x0F” instead of “0b00001111” and you can leave them in that format if you wish.
Writing a whole byte to a register is pretty straightforward, but it gets more complicated when you need to change only one of the bit-switches inside a control register. Then the standard approach is to first read out the register’s current settings, do some bit-math on that byte to affect only bit(s) you want to change, and then write that altered byte back into register’s memory location.
But bit-math syntax is one of those “devils in the details” that makes relatively simple code unreadable by beginners. The bit operators you absolutely must be familiar with to understand sensor scripts you find on the web are: the bitwise OR operator [|] , the bitwise AND operator [&], the left shift [<<] and the right shift [>>] operators. Fortunately there is an excellent explanation of how they work over at the Arduino playground, with a set of bit-math recipes in the quick reference section that let you reach into a byte of data and affect one bit at a time. Be sure to parenthesize everything when using bitwise operators because the order of operations can be counter-intuitive, and don’t worry if you have to look up the combinations every time because most people forget those details once they have their code working. I know I do.
Two particularly useful procedures:
x &= ~(1 << n); // AND inverse (~) forces nth bit of x to be 0. All other bits left alone
x |= (1 << n); // OR forces nth bit of x to be 1. All other bits left alone
And these let us add a third function to the standard set which will turn on or turn off one single bit switch in a sensors control register:
byte registerByte, result;
registerByte = i2c_readRegisterByte ( deviceAddress, registerAddress ); // load the current register byte
else { // when state = 0
registerByte &= ~(1 << bitPosition); // bitPosition now = 0
}
The ADXL345 accelerometer supports low power modes that use about 1/3 less power than the ‘standard’ operating modes. The sensor is not turned off, but the bandwidth is reduced somewhat, so the sensor responds more slowly to things like tap inputs. An example which sets the single bit enabling this low power mode might look like:
i2c_setRegisterBit( ADXL345_ADDRESS, ADXL345_BW_RATE, 5, 1 );
Many I2C sensors have power saving features like that which rarely get utilized. Note that bit position numbering starts with 0 and counts from the left OR the right hand side depending on the sensor manufacturer.
Some devices have control registers that are 16-bits wide. These get treated as a pair of 8-bit bytes that are read-from or written-to sequentially. You only have to specify the device & register address once at the beginning of the process because the sensors internal memory pointers get incremented automatically during the process.
This adds an extra wire.write step to the basic register writing operation:
Wire.beginTransmission(deviceAddress);
Wire.write(registerAddress);
Wire.write(MSB_registerData); // Send the “upper” or most significant bits
Wire.write(LSB_registerData); // Send the “lower” or least significant bits
Wire.endTransmission();
The MCP9808 is a common temperature sensor that uses 16-bit control registers. Setting “bit 8” of the CONFIG register to 1 puts the sensor into shut down mode between readings and setting that bit to 0 starts the sensor up again. (yes, that’s opposite to the usual on/off pattern…) The 8-bit limitation of the I²C bus forces us to retrieve the register in two separate bytes, so bit 8 of the 16 bits described in the datasheet ends up in the zero bit position of MSB.
A custom function shutting down the MCP9808 might look like this:
void mcp9808shutdown() //since we used defines, we did not pass any byte variables into the function
byte MSB, LSB;
Wire.requestFrom(MCP9808_i2cAddress, 2); //request the two bytes
Wire.write(MCP9808_REG_CONFIG);
Wire.write(MSB); // the one we modified
This ‘position x becomes position y’ translation is common stumbling block for beginners working with multi-byte registers – especially when you add reverse order position numbering into the mix. But there’s another gotcha with control registers that’s even more frustrating if you don’t catch it on your first pass through the datasheet: Sometimes there are special “write protection” registers that have to be set before you can change any of the other control registers, and these have to be changed back to their “protecting” state before those new settings take effect. You might not get any error messages, but nothing will work the way it should until you get the protection bits disabled and re-enabled in the right sequence. Fortunately less than 20% of the sensors I’ve worked with have this feature.
Another thing to watch out for are old code examples on the web that appear to be using integer variables to store device and memory register locations, with statements like Wire.send((int)(eepromaddress >> 8)); The I²C wire library only sends bytes/uint8_ts, but people got away with this (int) cast because it was being corrected behind the scenes by the library, which re-cast the value into a byte at compile time. The (byte) data type on Arduino is interchangeable with the (uint8_t) variables you see in most C++ coding tutorials.
2) Data registers
Unlike a control registers bank-of-switches, I think of data output registers as containers holding numbers which just happen to be stored in binary form. Since eight bits can only hold decimal system values from 0 to 255 you usually have to “re-assemble” larger sensor output values from bytes stored in consecutive memory locations. For sensors like the ADXL345 you can concatenate the two 8-bit bytes into one 16-bit integer variable by shifting the MSB left by 8 positions and merging in the LSB with a bitwise OR :
Wire.beginTransmission(deviceAddressByte); // the pointer setting transaction
Wire.write(registerAddressByte);
Wire.endTransmission();
Wire.requestFrom(deviceAddressByte,2); // request two bytes
LSB = Wire.read(); // byte from registerAddressByte
MSB = Wire.read(); // byte from registerAddressByte +1
int combined = (int)MSB; // MSB now in rightmost 8 bits of combined int
combined = combined<<8; // shift those bits to the left by 8 positions
combined |= LSB; // logical OR keeps upper bits intact and fills in rightmost 8 bits
Those steps are usually written in one single line as:
int combined = (((int)MSB) << 8) | LSB;
There are several other ways to combine bytes and some sensors send the MSB first – so you have to check the register map in the datasheet to know the order of the bytes that arrive from the output registers when you request multiple.
Now if you are thinking that looked too easy – you’re right! Most hobby market I2C sensors only have a 12-bit ADC, and since memory is a limited resource there are often status register bits mixed in with the data held in the MSB. Since these bits are not part of the sensor reading, you need to &-mask them away before you combine the MSB & LSB. It gets trickier when the sensor output can be a positive or a negative number because signed and unsigned integers are distinguished from each other by a special “sign” indicator bit, which can accidentally be turned into a “number” bit by bit shifting. (see: ‘sign extension’ in that bit math tutorial )
The temperature data output register in the MCP9808 is a good example of both of these issues:
Bits 15-13 (which become the top 3 bits of the upperByte in the code below) are status indicator flags identifying when high & low temp. alarm thresholds have been crossed. Bit 12 is a sign bit (0 for +ve temperature or 1 or -ve temps). The remaining bits 11-8 (=bits 3-0 of the upperByte) are the most significant 4-bits of the 12-bit integer representing the temperature.
So a sensor-specific approach to reading the temp. from an MCP9808 might look like this:
float TEMP_degC;
// spacer comment for blog layout
Wire.write(0x05); // and the temperature output register
Wire.endTransmission();
if ((UpperByte & 0b00010000) == 0b00010000) { // if sign bit =1 then temp < 0°C
UpperByte = UpperByte & 0b00001111; // mask away the SIGN bit
{
}
TEMP_degC =TEMP_Raw*0.0625;
Typically a data output register will continue to hold the last sensor reading until it is refilled with the next one. If your sensor takes a long time to generate this new reading (30-250 ms is typical, while some can take up to a second) and you read the registers before the new data is ready, you can end up loading the previous sensor reading by mistake. That’s where status registers come to the rescue.
3) Status registers
These tell you if if a specified type of event has occurred and I think of these registers as a set of YES/NO answers to eight different questions. The most commonly used status register is data ready [usually labeled DRDY] which sets a bit to 1=true when a new sensor reading is available to be read from the related output registers. Another common status register is one that becomes true if a sensor reading has passed some sort of threshold (like a low temperature alert, or a falling/tilt-angle warning).
A function to check the true=1/false=0 state of a single DRDY bit inside an 8-bit status register might look like this:
byte registerByte;
// You could use i2c_getRegisterBit to check the DRDY status bit with a do-while loop
Holding the processor captive in a status-bit-reading loop is very easy to do, but it is usually avoided unless you are trying to capture a series of sensor readings quickly. Most status register bits can be mapped to physical alarm output lines on the sensor module, and these can be used to trigger a hardware interrupt pin (D2 & D3) on the Arduino. This lets you to setup an interrupt service routine (ISR) which grabs that new reading even faster than a bit reading loop. And since hardware interrupts can be used wake a sleeping processor, the interrupt method also lets you put your data logger to sleep until something actually happens.
The only drawback to the ISR method is that the sequence of register settings you need to follow to create hardware alarms is another multi-step process to add to your sensor initialization code. The conceptual pattern is usually something like:
- Disable the sensor’s global interrupt control bit (if there is one)
- Enable the sensors triggering function (eg: a max. temperature alert)
- Load register(s) with the parameter value for that trigger (eg: 52.5°C)
- Turn on the status register that listens to that triggering function
- Map that status register bit to a hardware output line
- Re-enable the global interrupt control bit

This LSM303 combined accelerometer / magnetometer sensor has two alarm outputs in addition to DRDY. So you could map the Accelerometers DRDY signal to int1, and the Magnetometers DRDY to DRDY. Just to make life interesting with this sensor, the 3-axis output data registers are arranged in a different order on the magnetometer than they are on the accleerometer. This is typical for multi-sensor chips, which you handle like separate sensors even if they come in the same package – you can even put one to sleep mode while the other one is taking a reading.
Sensors can have many different status monitoring functions, but they usually have only one or two hardware alarm lines. So the status register -> hardware output mapping (step 5) listed above sometimes involves its own sequence of register settings. As example, the ADXL345 reads acceleration on three axes, and it has double-tap detection functions for each x,y,z direction. But the Arduino only has two incoming hardware interrupt lines. So generally speaking, you would map all three of those tap-detect status registers to the same outgoing alarm line on the sensor module, and then have the program figure out which axis actually triggered the alarm by reading the status registers later on. High & Low temperature sensor alerts are often mapped in a similar fashion because many breakouts only have one outgoing line: especially if the DRDY status register has been permanently connected to the only other physical alarm line.
A conceptual twist here is that most of the time, the hardware output actually moves the line LOW when the alarm is triggered, even if the status bit it’s mapped from is true=1=high when the actual event occurs. No matter what the status bit->alarm pattern is, any of the four possible interrupt triggers: HIGH, LOW, RISING & FALLING can be used to wake a sleeping 328p processor (though the datasheet states differently).
Another thing to watch out for setting your ISR to respond to HIGH/LOW levels rather than RISING/FALLING edges: Level based interrupts will keep triggering as long as that line is HIGH/LOW. This could cause a sketch to run extremely slowly until the interrupt handler is disabled in your program. Even old analog reed-switched based sensors can suffer from this type of issue, as its not uncommon for something like a wind sensor to stop spinning right where the magnet is holding the reed-switch closed. The thing that makes this choice somewhat tricky is that the most common type of sensor failure I see is one where the alarm stays on permanently. If you set your interrupt to respond to LOW, and the sensors starts self-triggering your event counters get pushed up to ridiculously large numbers – so it’s very easy to spot that failure in the data, and by the fact that the logger is usually kept awake till the batteries run dry. If your ISR responds to FALLING, your counts go to almost zero in the same situation, and depending on the phenomenon you are recording it could be very easy to miss that a sensor problem has developed.
For more information, there’s an excellent guide to interrupt handling over at the Gammon Forum. Probably the most important thing to keep in mind about using interrupts is that by default all interrupts are disabled once you are inside an interrupt subroutine so that the ISR can’t interrupt itself and create an infinite-recursion situation that over-runs the memory. But the I2C bus relies on interrupts to function, along with timers and other important things. So don’t try to change a sensor register while inside the ISR, just set a volatile flag variable and deal with resetting registers later in the main loop. The general rule of thumb is: “get in & get out ” as fast as possible, and I rarely have a sensor triggered ISR longer than this:
void INT1pinD3_triggered() { INT1_Flag = true; }
though sometimes I’ll also detachInterrupt(interrupt#) inside the ISR, to make sure it only fires once for things like button de-bouncing.
Status registers are usually latched, and have to be reset by the I²C master after they are triggered. DRDY registers are cleared by reading information from the data registers they are associated with. Most other status registers are cleared by reading the register’s memory location, which also turns off the hardware alarm signals that were mapped from them. This is different from control registers which always have to be explicitly over-written to with new information to change them. If you are waking up a sleeping data logger based on something like a high temperature alert, you usually read the status registers to clear those alarms before enabling interrupts and putting your logger into a power-down state. Threshold based alarms allow interesting things like burst logging.
In Summary:
A good register map, and the four generic functions I’ve described here
- i2c_readRegisterByte
- i2c_writeRegisterByte
- i2c_setRegisterBit
- i2c_getRegisterBit
Should be enough to get a typical I²C sensor running, and you can easily tweak those functions to make custom versions for reading 16-bit registers and/or to mask the cruft out of data pulled from mixed registers.

After testing an I2C sensor combination, I pot them in epoxy. Detailed instructions here.
Initializing an I²C sensor is a multi-step process and the correct order of operations is often poorly explained in the data sheet because they are usually written “in reverse”. Instead of a straightforward list saying “To get a reading from this sensor, do (1),(2),(3),(4), etc.” you find descriptions of the control register bits saying “before you set bit x in this register you must set bit y in this other control register”. When you look up that other control register you find that it too contains a sentence at the end saying “before you set bit y in this control register you must set bit z in this other control register”. So you have to work your way through the document, tracing all those links back until you find the things you were supposed to do first. Finding the “prime control bit” can be such a time consuming process that it’s not unusual for people who figure out the sequence to wrap it all up into a sensor library so they never have to look at that damn datasheet ever again.
But if you use those libraries, keep in mind that they are probably going to configure your sensor to run at the highest possible bit-depth & data rate, unnecessarily burning away power in applications like data logging which might only need one reading every fifteen minutes. So the majority of off-the-shelf sensor libraries should be seen as partial solutions, and you don’t really know what else your sensor is capable of until you read through the datasheet yourself. As an example there are IMU’s out there that will do Euler angle calculations if you simply turn on those functions with the right control register. But libraries for those chips sometimes enable the bare minimum data output functionality, and then do computational handstands to accomplish those gnarly (long) calculations on the Arduino’s modest µC.
In addition there can be useful sensor functions hidden in plain sight, because the datasheet tells you how to turn them on & off, but gives you no clue when to do so. An example here would be humidity sensors like the HTU21D which has an on-chip heating element to help the sensor recover from long periods of condensation, but no status alert that would let you do this automatically. You could just run the heater once a day, but there is also no indication how long the sensor would last if you did that – just some vague references to “functionality diagnosis”. But then some manufacturers (Freescale and Sensirion come to mind…) commit more than just sins-of-omission, breaking away with non-standard I²C bus implementations to lock in customers. The logic there is that if you have to buy the one great sensor that only they make, it’s easier to buy the other four sensors for your device from them as well, rather than juggling low-level protocol conflicts.
Another challenge when you are working with a new sensor is that Arduino’s C++ environment is not the same as vanilla C in some important ways. So many of the tutorials you find will describe methods that won’t work on an Arduino. Even when the code does compile, there are a number of different “styles” that are functionally identical when they pop out the other side of the compiler, so I’m still trying to wrap my head around the syntax that turns arrays into pointers when they get passed into functions. That’s why I didn’t mention I2C eeproms in a post about memory registers: almost every multi-byte read/write example out there for EEprom’s uses array/pointer/reference thingies. If you absolutely have to read a series of sensor output registers into an array with a loop, my advice is to just make it a global until you really know what you are doing. And don’t try to store numbers in a char array, because the “temporary promotion” of int8_t’s to 16-bit during some operations can bung up the calculations.
But now it’s time to bring this thing to a close. While I’m still thinking about stuff I wish I’d known earlier, it occurs that a good follow-on to this post would be one about techniques for post-processing sensor data. There are plenty of useful methods like Paul Badger’s digital smooth, and other code tricks like wrapping those functions in #ifdef #endif statements so those routines only get compiled when a sensor that actually needs them is connected to your logger.
That will have to wait for another day so for now I’ll just sign off with some links. Except for that last ranty bit, I’ve tried to stay out of the I²C handshaking weeds, because when you are up to your neck in bit banging, it’s easy to forget you were trying to measure the water level in a swamp. But if that’s your thing, there’s some more advanced I²C code examples over at the Gammon Forum, an in depth reference to the Wire library at the Arduino playground , and some troubleshooting tips over at Hackaday. Its also worth noting that I’ve used bit-shifting to extract bits, and concatenate 16-bit values from 8-bit registers. But you sometimes run into examples where people have uses structs & unions to do those tasks in a much more elegant way.
Addendum 2017-11-04

I wonder how many other sensors I could use this with? And if my pin-toggled oversampling method works on the ATtiny, this might provide better resolution than some commercial sensors; though I guess that would depend on how much I could squeeze into only 512 bytes of SRAM…
Somehow I always seem to run into a bunch of related material the day after I post something to this blog: There’s a cool little project over at Quad Me Up using ATtiny85 to turn an analog light sensor into an I2C slave device. AN4418 from Maxim explains how to use I/O extenders to connect a compact-flash (CF) cards to the I2C interface, which is something I never thought I’d see. And then theres AN10658 from NXP with a method for sending I²C-bus signals over 100m. My own tests with the I²C sensors just hanging off the Arduino only reached about 20m. Technoblogy has used this ATtiny approach to build an I2C SD card interface and an I2C GPS interface.
Addendum 2017-11-05
Koepel over at the Arduino forum pointed out that the IDE supports some handy macros like bitSet(), bitClear(), and bitRead() that could replace the bit math & masking functions I described above:
byte resisterData = Wire.read(); // byte from register
bitSet(resisterData, 6); // change bit 6 to a 1
These handy macros are particularly helpful when setting the internal behavior of the processor on the Arduino board because all the bits in those registers have names that the IDE can translate into actual numbers:
bitSet (DIDR0, ADC2D); // disable input buffer on A2 by setting ADC2D bit in register DIDR0
Another highly useful trick is to to use bit_is_set or bit_is_clear macros to poll the value of one of your processors flag registers inside a while loop to create short-duration conditional delays while some process occurs:
while (bit_is_set(ADCSRA,ADSC)); // waits here for ADC conversion to complete
There’s also word(h , l) to combine two bytes, or highByte() and lowByte() to divide 16-bit variables into 8-bit two pieces. These macros may not travel well outside the Arduino IDE, so most programmers avoid using them, preferring to write out the explicit code.
So bitSet replaces x |= (1 << n); and bitClear can be used to replace x &= ~(1 << n); in in the standard I2C functions I described in the post. Many programmers do not like the code underlying these kinds of compiler substituted macros, so the bit math expressions are far more commonly used because they are generally faster to execute. The AVR can only shift bit positions by one bit per clock cycle (so <<n, takes n clock cycles) but that’s still faster.
There is one other bit-math expression I use frequently, when I want to toggle the state of I/O pins, for example: PORTD ^= B00001000; (toggles the state of only bits with a “1” on the right hand side of the expression) That is a special use case for rapid port switching on the Arduino, rather than for sensor register bits.
Addendum 2017-11-06
I’ve also just found out that there are a small number of sensors there that require a ‘false’ modifier to be used at the end of an I²C transaction: Wire.endTransmission(false); This is called a repeated start, and the I2C master does not release the bus between writing the register-address and reading data with Wire.requestFrom(); The sensor responds to the I²C address with an acknowledge at the begin of the I²C transaction, and to each databyte that is written to the sensor, so the error code returned by endTransmission can still be used because it is a test if the I²C address was acknowledged by the sensor.
And there was another I²C quirk mentioned at the Gammon Forum:
“You can’t rely on the slave necessarily sending the number of bytes you requested. If you request 10 bytes, Wire.requestFrom() will return 10, even if only 5 have been sent. For the slave to terminate the communication early (ie. after sending less than the requested number of bytes) it would have to be able to raise a “stop condition”. Only the master can do that, as the master controls the I2C clock. Thus, if the slave stops sending before the requested number of bytes have been sent, the pull-up resistors pull SDA high, and the master simply receives one or more 0xFF bytes as the response…It does not help to use Wire.available() because that too will return the number of bytes requested.”
Another little gem about the I2C interface on the Atmel chips:
“The Twin Wire Interface is virtually identical to the I2C bus. This is actually the bus that the Arduino uses, TWI was developed when the I2C bus was not open source and Atmel did not want to risk a trade name violation. The only major difference between TWI and I2C is that TWI does not support an advanced technique called clock stretching.”
Addendum 2017-11-08
On my page about the DS3231 rtc I describe how to power that I²C chip from a digital pin during bus communication. That trick only works because the chip was designed to gracefully fail over to a backup coin-cell power supply. With other I²C sensors a leakage current might flow into the sensor through the pullup resistors, so you would have to power the bus pullups with the same digital pin to avoid this. And since the internal pullup resistors are enabled by default in the Wire library, you have to disable I²C before you could pin power that I²C device. Also don’t try to de-power a whole module with decoupling capacitors through a digital output pin as that creates big current spikes and really needs proper switching with a PNP transistor of p-channel FET. 99.99% of the time its better to simply find a sensor with a really low sleep current sleep state that you can enter by setting a control register. The best sensors are ones that automatically go into these low current standby-states whenever they detect no traffic on the I2C bus: then you don’t have to do anything.
Another thing I discovered while working with that RTC was that it had a Wire.begin() call hidden in the library, but I was already starting the I²C bus normally during setup. So without knowing it the I²C hardware was being initialized a second time. As the I²C peripheral registers are set to the same value as in the first Wire.begin() call nothing bad happened. However I can see where it might get’s problematic if you call Wire.begin() accidentally because it was buried inside some sensor library while you were running a data transfer, and the hardware is re-set to an idle state.
Addendum 2017-11-09
Most of us are familiar with trying out different libraries to drive a sensor, but I’d be remiss if I didn’t mention that there are also some alternatives to Wire library for I²C. The one that gets the best reviews is the I2C Master Library developed by Wayne Truchsess at DSSCircuits. This lib has a faster throughput and a significantly smaller code size: the original Wire library adds about 796 bytes to your sketch when included whereas Wayne’s I²C library only adds 140 bytes. And it has built-in commands that replicate all of the functions I described in this post. For 16 bit registers Wayne points out:
“Technically when sending bytes to a slave device there is no difference between data and an address. In other words let’s say you have a three byte address and three bytes of data. You could use the write(address, regaddress, *data) by making the first byte of your multibyte address equal to regaddress and then combine the rest of the address and data together into *data.”
and that’s equally true with the wire library. The memory savings alone would be worth exploring, but perhaps I²C Master library’s most compelling feature is a ‘TimeOut’ parameter for all bus coms, which could keep your logger from getting stuck in a while-loop if one of your sensors goes AWOL, though I wonder if it still has the 0xFF problem mentioned above, if the sensor sends less than you requested? And there are lots of other I2C libraries to explore, and even some updated versions of the lib from DSS.
Addendum 2017-11-10
I thought using an ATtiny to convert an analog sensor into an I²C device was a neat trick. But it seems that Andreas Spiess has taken the idea to a new level with three HC-SR04’saccessible through on a single AT. His youTube video #42 with three Ultrasonic Sensors for Arduino walks through the process, with a vocal track that leaves you thinking Werner Herzog has started doing maker videos. I am impressed with what people achieve using those little processors. The basic idea of reading an analog signal and turning it into PWM output is common to both amplifiers and sensor applications like light->frequency converters.
Addendum 2017-11-13
The IDE compiler has an annoying quirk when it runs into Wire.requestFrom in those I2C register routines because the compiler throws up warning messages whenever it feels it has to resolve an ‘ambiguous’ call: (click to enlarge)
Turns out that requestFrom has two different implementations, one that can take int arguments, and one that takes uint8_t arguments. If you put in something which has no type like a number (or something you declared with a #define) the compiler has to decide which implementation to use. In the case shown above it chose to use the (int,int) flavor even though device address was specified as uint_8 at the start of the function.
Anyway, to make those warnings disappear, simply cast the two parameters in Wire.requestFrom to either (uint_8) or (int):
And all those compiler warnings will disappear.
Addendum 2017-12-14
Single I2C/SMBus Address Translator for those times when you have an unavoidable sensor bus address conflict. Or you can use an I²C multiplexer like the TCA9548A over at Adafruit which will let you use one I²C address to talk to the multiplexer and tell it which lane you want to enable.
Addendum 2019-06-11: How fast is the I2C bus?
There’s different ways to think about this question, and most sources quote low-level numbers that make it hard to see the forest for the trees. From the perspective of driving an I2C sensor what you usually want to know is how many times can I capture readings before I have a situation where the bus can’t keep up? (in those cases you usually switch to SPI sensors)
Nick Gammons I2C page has logic analyzer shots showing the timing for an address byte and single data byte transaction taking ~0.2 milliseconds. A little further down the page he shows another transaction sending the address & two bytes to an 24LC256 eeprom which took about 0.3 ms at the 100 Khz default. And at Saleae.com I found perhaps the ‘most typical’ type of sensor transaction:
You capture a reading from the TMP102 temp sensor shown above with the standard I2C sequence:
1) set the devices memory pointer address to the output register
2) read two bytes of data from the sensor
The sequence takes just under 0.5 ms, so you could achieve ~2000 of these transactions per second. Add another handshake to ‘trigger each conversion’ and you cut throughput to around 1000 ‘complete sensor transactions’ per second. The TMP102 needs about 25ms to actually take each reading, so the bus is 25x faster at the 100Khz default speed. However there are plenty of other sensors with output data rates that can approach, or exceed, that 1000 samples per second, and if you are juggling a few of those for a balancing robot or a drone you can create a bottleneck – and remember the wire library is also preventing your processor from doing anything else for that time. Fortunately it’s easy to make the bus 4x faster with the TWBR command. And on 16Mhz Arduinos, I can usually push that to 800 Khz for faster sensors if my wires are short enough.
The DS3231 RTC module we use on our loggers has 4k7 ohm pull-up resistors on the SDA & SCL lines & the Pro Mini adds internal 50k pull ups when the wire library is enabled. I2C sensor modules usually add another 10k each, so our ‘net pullup resistance’ on the I2C bus wires is usually: 50k // 4k7 // 10k = ~3k. With a 3.3v rail that means the devices draw 3.3v / 3k = 1 mA during communication which is fairly normal ( 3mA is max current allowed by the spec) for total wire lengths below 1m. Longer wires and multiple sensors/devices add capacitance to the bus and may require a lower frequency for stable communications. You can change the speed of the I2C bus from its 100kHz default with the TBWR= #; command and the resulting frequency can be derived from this formula:
TWBR = ((mainCPUclockSpeed / BusFrequency) – 16) / 2; (assumes default prescalar of 1)
You can set any frequency where that formula generating an integer between 0 and 255 however a given CPU frequency cannot generate all possible bus speeds. And the I2C device(s) will set restrictions on the maximum allowed -> the device with the lowest frequency sets your safe upper limit.
The I2C bus speeds you typically see listed for sensors are 100KHz & 400KHz. The RTC module we use in the Cave Pearl loggers has a 100kHz eeprom on board but the DS3231 chip is good to 400 kHz, so on builds where I’m not using the eeprom I will bump the clock up to 400 as most sensors also support the faster speed. Doing so is a risk because there’s no guarantee that the 100kHz device will not misbehave when exposed to 400kHz traffic – anything from NACKs to bus hangs are possible. It’s also worth noting that on 8Mhz ProMini style Arduinos, pushing the bus clock up to 400kHz (with TWBR=2;) shortens t_low to 1μs, which is below the 1.3 microsecond minimum twi spec. So again you have to test your system thoroughly, and if devices fall of the bus at 400 you can step back to 200kHz. (TWBR = 12;)
So far we’ve gotten away with the faster bus however MOST of the time I actually use TWBR settings to slow down the bus to 50 kHz or even 25 kHz (TWBR = 152; @8MHz) when my wires get longer than 1m, or when multiple sensors have started to cause communication errors. There is no minimum clock frequency in the I2C spec, but regardless of the clock speed the rise time for signals on long cables can’t exceed 1000ns (and the fall time can’t be longer than 300ns – but that’s rarely an issue). Changing to more aggressive 2k2 pullup resistors also helps when you need to hang an I2C sensor off of long wires. We’ve successfully pushed that out to 20m on some of our builds.
Addendum 2020-05-21: Using I2C sensor alarms to interrupt processor sleeping
I just put together a post on the ADS1115 module, which, while it’s not a sensor per se, still provides a good example of how I use ‘DATA ready’ alarms to let me wake & sleep the processor to save power in logging applications. Many slow I2C sensors have similar ALRT/RDY output pins, so the do{ } while (condition); loops shown in that post are transferable to other sensor modules.
Addendum 2021-01-07: Resolving I2C address conflict
Another interesting solution to running multiple I2C sensors with the same address:
“Our solution was to use I/O lines on the microcontroller to force the SDA lines high on the devices that we don’t want to address, while the I/O line for the device we’re targeting is set as input (high impedance). This means that only the targeted device matches it’s I2C address and the others ignore any subsequent data.”
Addendum 2023-12-01: Mini Breadboards for fast I2C sensor swapping
The easiest way to make your build capable of supporting multiple different configurations of I2C sensors is to add mini breadboards to the design. We added this flexibility to the 2-module classroom data loggers with 3D printed holder rails. The only limitation is that leaves only one extra connection row for the sensor alarm or address configuration.